给定一个单词数组和一个长度 maxWidth,重新排版单词,使其成为每行恰好有 maxWidth 个字符,且左右两端对齐的文本。
你应该使用“贪心算法”来放置给定的单词;也就是说,尽可能多地往每行中放置单词。必要时可用空格 ' '
填充,使得每行恰好有 maxWidth 个字符。
要求尽可能均匀分配单词间的空格数量。如果某一行单词间的空格不能均匀分配,则左侧放置的空格数要多于右侧的空格数。
文本的最后一行应为左对齐,且单词之间不插入额外的空格。
说明:
- 单词是指由非空格字符组成的字符序列。
- 每个单词的长度大于 0,小于等于 maxWidth。
- 输入单词数组
words
至少包含一个单词。
示例:
输入:
words = ["This", "is", "an", "example", "of", "text", "justification."]
maxWidth = 16
输出:
[
"This is an",
"example of text",
"justification. "
]
示例 2:
输入:
words = ["What","must","be","acknowledgment","shall","be"]
maxWidth = 16
输出:
[
"What must be",
"acknowledgment ",
"shall be "
]
解释: 注意最后一行的格式应为 "shall be " 而不是 "shall be",
因为最后一行应为左对齐,而不是左右两端对齐。
第二行同样为左对齐,这是因为这行只包含一个单词。
示例 3:
输入:
words = ["Science","is","what","we","understand","well","enough","to","explain",
"to","a","computer.","Art","is","everything","else","we","do"]
maxWidth = 20
输出:
[
"Science is what we",
"understand well",
"enough to explain to",
"a computer. Art is",
"everything else we",
"do "
]
code:
# [68] 文本左右对齐
#
# @lc code=start
class Solution:
def fullJustify(self, words: List[str], maxWidth: int) -> List[str]:
lens=len(words)
rev=list()
nowlens=0
start=0
i=0
while i<lens:
wordlen=len(words[i])
nowlens=nowlens+wordlen
#print(i,nowlens)
if nowlens<maxWidth:
nowlens+=1
i=i+1
#print(i)
#print(nowlens)
elif nowlens>maxWidth:
nowlens=nowlens-wordlen-1
reslens=maxWidth-nowlens
m=(i-1-start)
if m==0:
mid=""
mid=mid+words[start]+(maxWidth-len(words[start]))*" "
rev.append(mid)
#print(reslens,reslens//(i-1-start),reslens/(i-1-start))
elif reslens//(i-1-start)==reslens/(i-1-start):
mid=""
x=reslens//(i-1-start)+1
for j in range(start,i-1):
mid=mid+words[j]+x*" "
mid=mid+words[i-1]
rev.append(mid)
else:
other=reslens%(i-1-start)
mid=""
x=reslens//(i-1-start)+1
print(x,other)
for j in range(start,i-1):
if other>0:
mid=mid+words[j]+x*" "+" "
other-=1
else:
mid=mid+words[j]+x*" "
mid=mid+words[i-1]
rev.append(mid)
nowlens=0
start=i
elif nowlens==maxWidth:
mid=""
for j in range(start,i):
mid=mid+words[j]+" "
mid=mid+words[i]
rev.append(mid)
start=i+1
nowlens=0
i=i+1
if start<lens:
mid=""
for j in range(start,lens):
mid=mid+words[j]+" "
mid=mid[:-1]
other=maxWidth-len(mid)
mid=mid+other*" "
rev.append(mid)
return rev
# @lc code=end
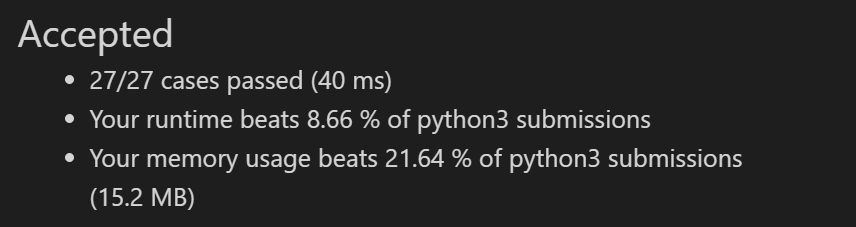